PA W2 - C Programming Language Basic
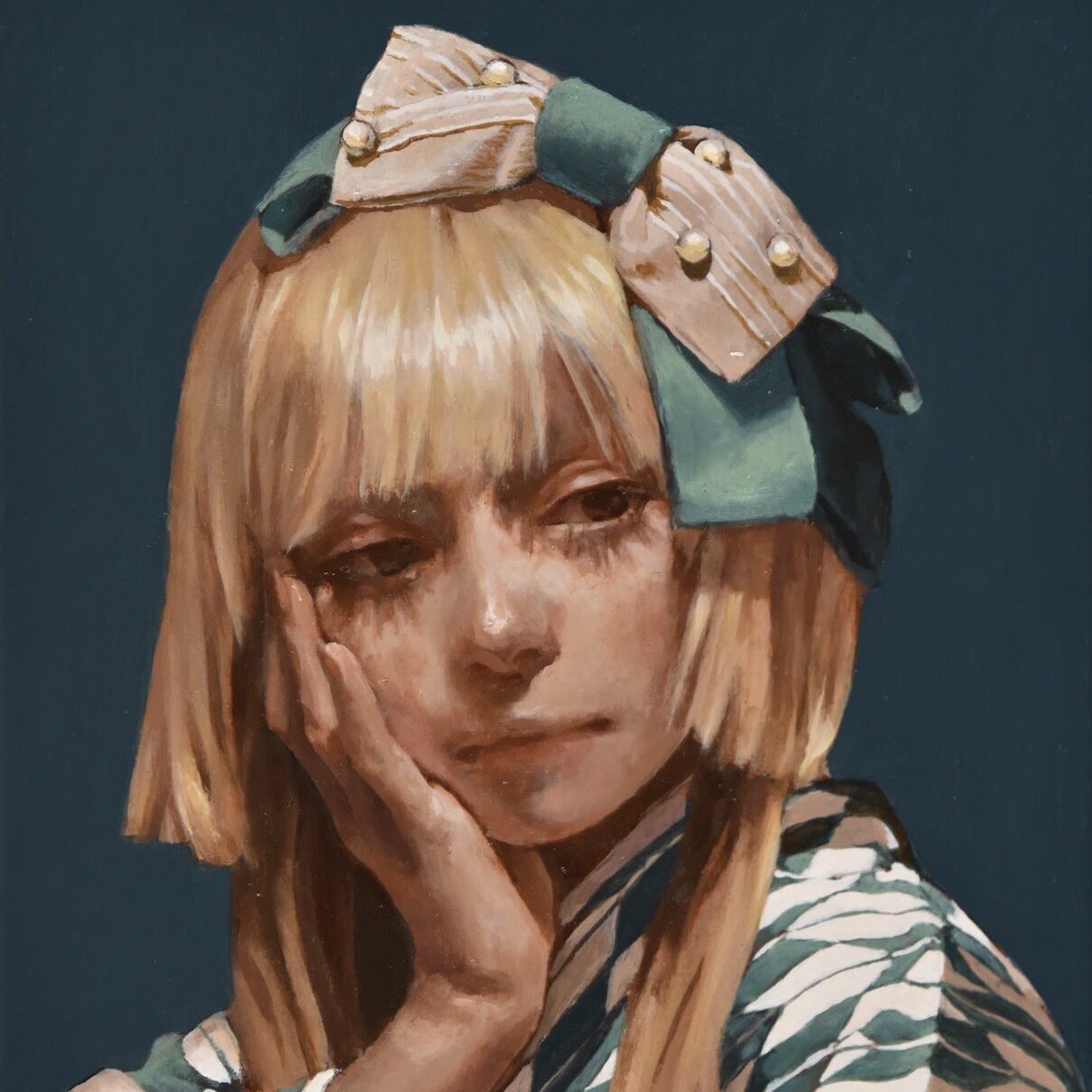
Warning
If someone is reading this blog, please be aware that the writer did not consider the experience of the other readers.
After all, the most important part is about writing things down for better memorization.
C programming language used in PA
- How to generate an executable file from a C source file?
1 | .c --precompile--> .i --compile--> .s --assemble--> .o --link--> .out |
Precompile
What are precompile behavior?
For example, these are precompile behavior:
1 |
|
include
Normally, head files do not contain the definition functions, instead, they include the declaration of the functions.(and some variables)
the declaration of the standard library function
printf
:1
int printf(const char *restrict format, ...);
if we declare this
printf
function before we invoke it, the program will run well even without includingstdio.h
:1
2
3
4
5
6int printf(const char *restrict format, ...);
int main(int argc, char *argv[]) {
printf("%d\n", a);
return 0;
}Thus, including head files are essentially copying the content inside the head files to the source code.
- What’s the differences between these two lines of code?
1
2 - The answer is: They are actually the same, except that they would search different path for the required head file.
- We can see the search list by adding
--verbose
flag to gcc:
- We can also add search path manualy by adding telling gcc the path with
-I
flag:1
gcc --verbose test1.c -I /home/last/Coding/Cpp/test
- We can see that
/home/last/Coding/Cpp/test/
is added to the search list. (as the first one)
#if
- What would this piece of code output?
1 |
|
The answer is
Yes
.But why? What exactly is
aa == bb
?Actually,
aa
andbb
are marcos. As shown in the code, these two macros are both not defined, thus making them equal as they are bothnull
.After precompilation is done, the code in the false branch of the if statement will be dropped.
#define
This statement is for defining macros. Macros will be expand when precompiling, which is basically copying and pasting again, just like the head file.
How to destroy an OJ? LMAO
From the picture above we can see that, macros can call(rely) on each other. It would be pretty common to see multiple macros calling each other in the source code of PA.
Macros can also be used to hide some keywords from inspection.
1 |
|
X-macro
1 |
|
- The output will be like:
1 | Hello, Tom! |
- We can see that
NAMES(PRINT)
calls the functionPRINT
for four times with different parameters each time:
1 | NAMES(PRINT) --> PRINT(Tom) PRINT(Jerry) PRINT(Tyke) PRINT(Spike) |
- From above we can see that macro provides a way for the programmer to define some sort of customized ‘function’ or ‘variable’, which is called meta-programming.
- It is very flexible and easy to use, but at the same time, it will reduce the readability of the code to some extend.
Compile && Assemble
- This step is mainly about translating the C programming language to the assembly language.
- For example, a simple function like:
1 | int foo(int n) { |
1 | gcc --assemble 4test-1.c |
will be translate to:
1 | 4.file "4test-1.c" |
- This rough file is hard to understand. But if we remove the parts which are unrelated to the core logic of the program, it will become this:
1 | foo: |
- Still hard to read for human, lets replace all of the variables with their names in the source code:
1 | foo: |
- Then, make it a pseudocode:
1 | foo: |
Link (Static Link)
- The file containing the assembly language translated from the
foo
function is not able to run yet, since it’s missing amain
function, the entry point. - Let’s quickly define a
main
function in a separate file.
4test-2.c:
1 |
|
- AWAK, this source file will also be translated to assembly language by gcc. After that, gcc will link the two .o file together, producing the final
a.out
executable file.
From a Memory Perspective
- Why would this code report a segmentation fault?
1 | int main(int argc, char* argv[]) { |
- From the code we can see that, this program is trying to write value
1
to a special memory address. - The thing is that, the memory block which the program can read or write is limited, mostly the part which allocated to the program by the OS.
- This program is trying to write stuff into a memory address which does not ‘belong’ to it, which causes a segmentation fault.
Understanding Pointer
- Let’s explore the following code:
1 |
|
- It might be complicated when taking a first look, so take another look on this picture, which analyze the ‘pointing’ relationship between these pointers and variables:
- From the picture we can clearly see that, pointer
a
is pointing to the address which stores an address pointing to an address pointing to the first element ofargv
. first
is pointing to the first character of the first element ofargv
, which is a string, AKA, a character array.ch
is the first character of the first element ofargv
, which is …
End of the First Day…
- Title: PA W2 - C Programming Language Basic
- Author: Last
- Created at : 2024-06-15 10:57:09
- Link: https://blog.imlast.top/2024/06/15/nju-pa-c1-md/
- License: This work is licensed under CC BY-NC-SA 4.0.